In today’s fast-paced digital world, app performance is crucial for user satisfaction and retention. Slow, unresponsive apps can quickly lead to user frustration and abandonment. This article will explore various techniques and best practices for optimizing your code to enhance app performance.
Understanding the Importance of Code Optimization
Code optimization is the process of modifying your application to make it more efficient without changing its functionality. The benefits of optimization include:
- Faster execution times
- Reduced resource consumption
- Improved user experience
- Lower operating costs
- Better scalability
Key Areas for Code Optimization
1. Algorithmic Efficiency
The foundation of any performant application lies in its algorithms. Efficient algorithms can significantly reduce processing time and resource usage.
Tips for Algorithmic Optimization:
- Choose appropriate data structures (e.g., HashMaps for fast lookups)
- Implement efficient sorting algorithms (e.g., QuickSort for large datasets)
- Use dynamic programming to avoid redundant calculations
- Optimize time complexity, aiming for O(n) or O(log n) where possible
2. Memory Management
Proper memory management is crucial for maintaining app performance, especially on mobile devices with limited resources.
Memory Optimization Techniques:
- Implement object pooling for frequently used objects
- Use weak references to prevent memory leaks
- Optimize image loading and caching
- Dispose of unused resources promptly
3. Database Optimization
For data-driven applications, database operations can often be a bottleneck. Optimizing these operations can lead to significant performance improvements.
Database Optimization Strategies:
- Index frequently queried columns
- Use database normalization judiciously
- Implement query caching
- Optimize SQL queries (e.g., avoid SELECT *)
- Consider using NoSQL databases for certain use cases
4. Asynchronous Programming
Leveraging asynchronous programming can prevent UI freezes and improve the overall responsiveness of your application.
Asynchronous Best Practices:
- Use async/await patterns for I/O-bound operations
- Implement background threading for CPU-intensive tasks
- Utilize callback mechanisms to handle long-running operations
- Consider reactive programming paradigms (e.g., RxJava, Combine)
5. Network Optimization
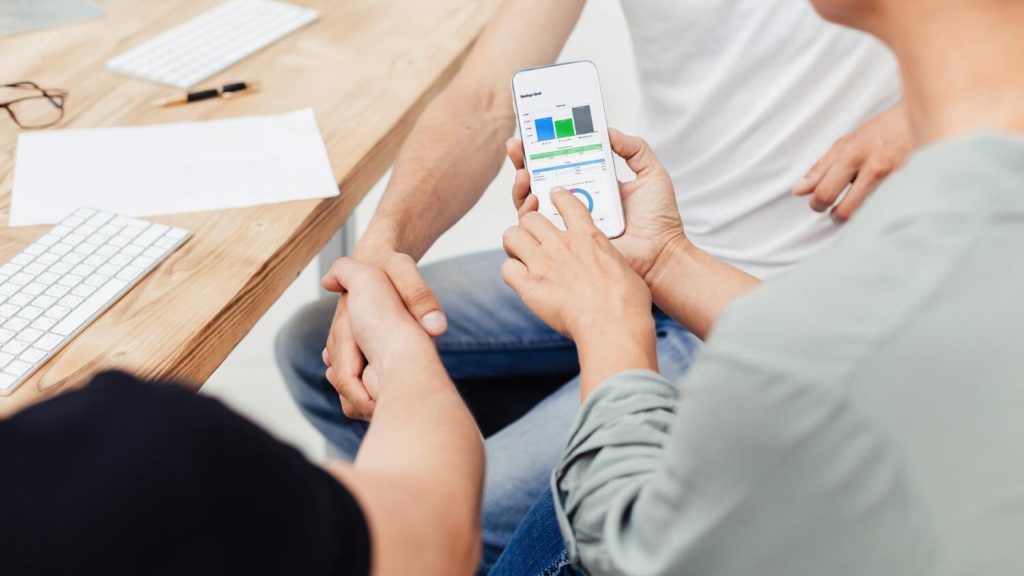
For apps that rely heavily on network operations, optimizing these processes is crucial for performance.
Network Optimization Techniques:
- Implement efficient data serialization (e.g., Protocol Buffers)
- Use compression for data transfers
- Implement proper caching strategies
- Optimize API calls (e.g., batch requests, pagination)
- Use efficient protocols (e.g., HTTP/2, WebSocket for real-time data)
6. Code-Level Optimizations
Sometimes, small changes in your code can lead to substantial performance improvements.
Code-Level Optimization Tips:
- Avoid unnecessary object creation
- Use StringBuilder for string concatenation in loops
- Implement lazy loading for resource-intensive components
- Optimize loops (e.g., break early when possible)
- Use appropriate scope for variables
7. UI Rendering Optimization
A smooth and responsive UI is critical for user perception of app performance.
UI Optimization Strategies:
- Implement view recycling in list views
- Use hardware acceleration when appropriate
- Optimize layouts (e.g., flatten view hierarchies)
- Use appropriate view types (e.g., ConstraintLayout for complex layouts)
- Implement efficient animations
Tools for Performance Analysis
To effectively optimize your app, you need to identify bottlenecks. Several tools can help with this process:
- Profilers: Use built-in profilers in IDEs like Android Studio or Xcode to analyze CPU, memory, and network usage.
- Analytics Tools: Implement crash reporting and performance monitoring tools like Firebase Performance Monitoring or New Relic.
- Network Inspectors: Use tools like Charles Proxy or Wireshark to analyze network traffic.
- Database Query Analyzers: Utilize database-specific tools to optimize queries and indexes.
Best Practices for Ongoing Optimization
Optimization is not a one-time task but an ongoing process. Here are some best practices to maintain optimal performance:
- Regular Code Reviews: Conduct peer reviews focusing on performance implications.
- Continuous Integration/Continuous Deployment (CI/CD): Implement automated performance tests in your CI/CD pipeline.
- Performance Budgets: Set performance targets and regularly measure against them.
- User Feedback: Pay attention to user reports of performance issues.
- Stay Updated: Keep your libraries, frameworks, and development tools up-to-date.
Conclusion
Improving app performance through code optimization is a multifaceted process that requires attention to various aspects of your application. By focusing on algorithmic efficiency, memory management, database operations, asynchronous programming, network optimization, code-level improvements, and UI rendering, you can significantly enhance your app’s performance.
Remember that optimization is an iterative process. Continuously monitor your app’s performance, identify bottlenecks, and apply these optimization techniques. With persistent effort and attention to detail, you can create a high-performing app that provides an excellent user experience and stands out in today’s competitive digital landscape.